April 8th, 2021 1 Minutes
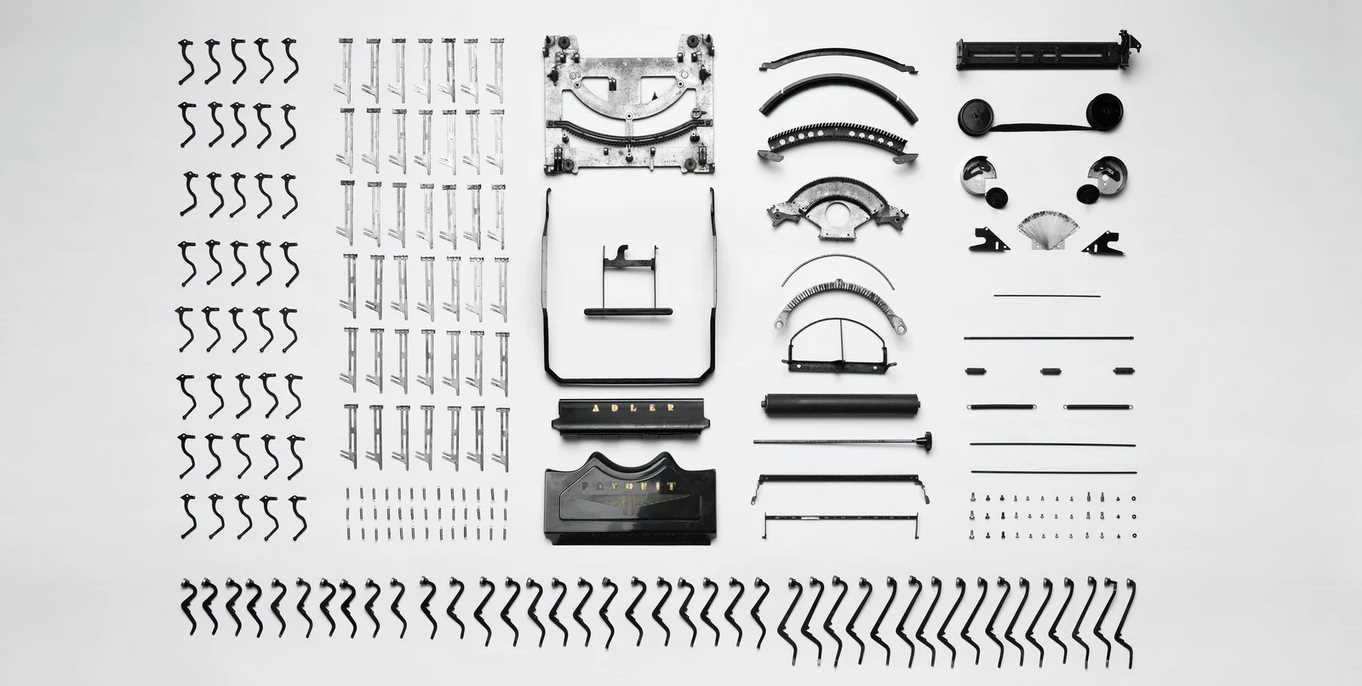
I've been using C# for about a decade now, and every now and again I discover something that surprises me. This week it's the ability to deconstruct as we do in Javascript (and I'm not talking about using Tuples!).
Below is a simple example of deconstruction taking place to draw out the power, and defence property for our Trex object,
const trex = {
statistics: {
power: 10,
defence: 2
},
name: "T-Rex",
};
const { power, defence } = trex.statistics;
console.log(`Power ${power}, Defence ${defence}`);
//Power 10, Defence 2
//Better than doing:
//const power = trex.statistics.power;
//const defence = trex.statistics.defence;
As Mozilla's definition states,
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables - Destructuring assignment.
It's a powerful syntactical sugar, especially in the scenarios where you have a nested object with long names. As deconstruction can lead to cleaner, more readable code its uses are great on large object types. Now let's take a look at the same thing but in C#,
namespace deconstruction
{
public record Statistics(int Power, int Defence);
public class Trex
{
public Statistics Statistics;
public string Name;
public Trex()
{
Name = "T-Rex";
Statistics = new Statistics(10, 5);
}
// Return the first and last name.
public void Deconstruct(out int power, out int defence)
{
power = Statistics.Power;
defence = Statistics.Defence;
}
}
}
Ok admittedly it's not as elegant as its Javascript counterpart as we need to define what we want to deconstruct upfront as well as have a function for each combination 😬! but it's still got its uses... check it out,
using System;
namespace deconstruction
{
class Program
{
static void Main(string[] args)
{
var trex = new Trex();
var (power, defence) = trex;
Console.WriteLine($"Power: {power}, Defence: {defence}");
//Power: 10, Defence: 5
//Better than doing:
//var power = trex.statistics.power;
//var defence = trex.statistics.defence;
}
}
}
Who knows what other hidden gems 💎 lie buried with the Microsoft docs!